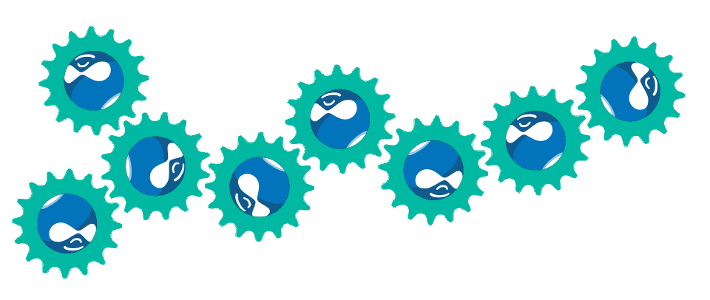
Creating a duplicate of an entity
Creating a duplicate of an entity is easily done via the entity API method Entity::createDuplicate(). This is a convenient method if the goal is to clone an entity into a new entity, as all identifiers of the previous entity get unset when using this method.
$nid = 5; $entity = \Drupal::service('entity_type.manager')->getStorage('node')->load($nid); // Use dependency injection instead if in class context. $duplicate = $entity->createDuplicate(); $duplicate->save();
Cloning data into an existing entity
However partially or fully cloning data into an existing entity is less straight forward (and rightfully so). Still, the ability to do so can be useful
- in custom migration scripts where we want to overwrite old entities without creating new ones,
- in cases where we need to overwrite the old entity as other internal or external data may reference it and creating a new entity would break these references.
The second case could be an entity reference field referencing the old entity in question (this could be technically solved by reassigning the reference), but it could also be 3rd party software referencing the old entity, which would complicate things.
This article is going to demonstrate a couple of possible ways of cloning entity data into existing entities.
Cloning field data 1:1
$source_nid = 5; $destination_nid = 6; // Use dependency injection in class context instead. $source = \Drupal::service('entity_type.manager')->getStorage('node')->load($source_nid); $destination = \Drupal::service('entity_type.manager')->getStorage('node')->load($destination_nid); foreach ($source->getFields() as $name => $field) { $destination->set($name, $field->getValue()); } $destination->save();
Importing new data only
To import new field data without overwriting existing data, just check if the destination field is empty before cloning into it like so:
foreach ($source->getFields() as $name => $field) { if ($destination->get($name)->isEmpty()) { $destination->set($name, $field->getValue()); } }
Putting it all together
A nifty method that could be used to clone field data of entities into other existing entitites could look like this:
/** * @param \Drupal\Core\Entity\Entity $source * @param \Drupal\Core\Entity\Entity $destination * @param string $mode * Can be 'keep', 'overwrite' and 'clone'. * @param array $skip_fields * An array of fields not to be cloned into the destination entity. */ public function cloneFields(Entity $source, Entity &$destination, $mode, $skip_fields = []) { foreach ($source->getFields() as $name => $field) { // In this case clone only fields and leave out properties like title. // Leave out certain fields. switch ($mode) { // Import only those fields from source that are empty in destination. case 'keep': default: if (!$destination->get($name)->isEmpty()) { continue 2; } break; // Import field data from source overwriting all destination fields. // Do not empty fields in destination if they are empty in source. case 'overwrite': if ($source->get($name)->isEmpty()) { continue 2; } break; // Import field data from source overwriting all destination fields. // Empty fields in destination if they are empty in source. case 'clone': break; } $destination->set($name, $field->getValue()); } } $destination->save(); }
There you go. Make sure to comment below in case of questions or if you know a better way of doing the above.
Comments
Thanks to this post, I was able to solve a problem that had no solution online: the ability to programmatically create a translation with all the fields of the original node. I scoured for solutions and found none, but thanks to your suggestion on iterating over fields, I was able to do it. Huge thank-you!
Here's the basic code that did it. I'm sure it needs tweaks as I'm skipping all non-fields except for path, but for now it's getting the job done!
You are welcome, I'm glad it helped!
Thank you for this. I am new to Drupal and learning. Would this be possible to use this on a taxonomy term where one wishes to copy all the content to another existing term?
Yes, that should work as a taxonomy term in Drupal 8 is a fieldable entity. You should be able to use this method without alterations. Maybe apart from not using the condition if (strpos($name, 'field') === 0
Add new comment