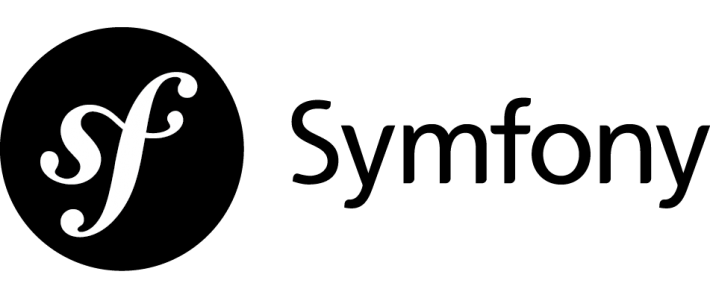
Occasionally I find myself needing plugin-like functionality, where users/downstream can throw a class into a folder and expect it to work. My script is supposed to find and instantiate these plugins during runtime without keeping track of their existence.
In a regular Drupal module, one would usually use the plugin architecture, but that comes with its overhead of boilerplate code and may not be the solution for the simplest of use cases.
Many class finder libraries rely on get_declared_classes() which may not be helpful, as the classes in question may not have been declared yet.
If you are on a Drupal 8/9 installation and want to use components already available to you, the Symfony (file) Finder can be an alternative for finding classes in a given namespace.
Installing dependencies
Ouside of Drupal 8/9, you may need to require this library in your application:
composer require symfony/finder
A simple example
use Symfony\Component\Finder\Finder; class PluginLoader { /** * Loads all plugins. * * @param string $namespace * Namespace required for a class to be considered a plugin. * @param string $search_root_path * Search classes recursively starting from this folder. * The default is the folder this here class resides in. * * @return object[] * Array of instantiated plugins */ $finder = new Finder(); $finder->files()->in($search_root_path)->name('*.php'); foreach ($finder as $file) { try { $plugins[] = new $class_name(); } catch (\Throwable $e) { continue; } } } return $plugins ?? []; } }
Usage
$plugin_instances = PluginLoader::loadPlugins('\Some\Namespace');
This is just an abstract catch-all example with a couple of obvious problems which can be circumvented when using more specific code.
In the above example, the finder looks for all files with the .php extension within all folders in a given path. If it finds a class, it tries to instantiate it. The try-catch block is for it to not fail when trying to instantiate non-instantiatable classes, interfaces and similar.
The above can be improved upon by making assumptions about the class name (one could be looking for class files named *Plugin.php) and examining the file content (which the Finder component is capable of as well).
Let me know of other simple ways of tackling this problem!
Add new comment